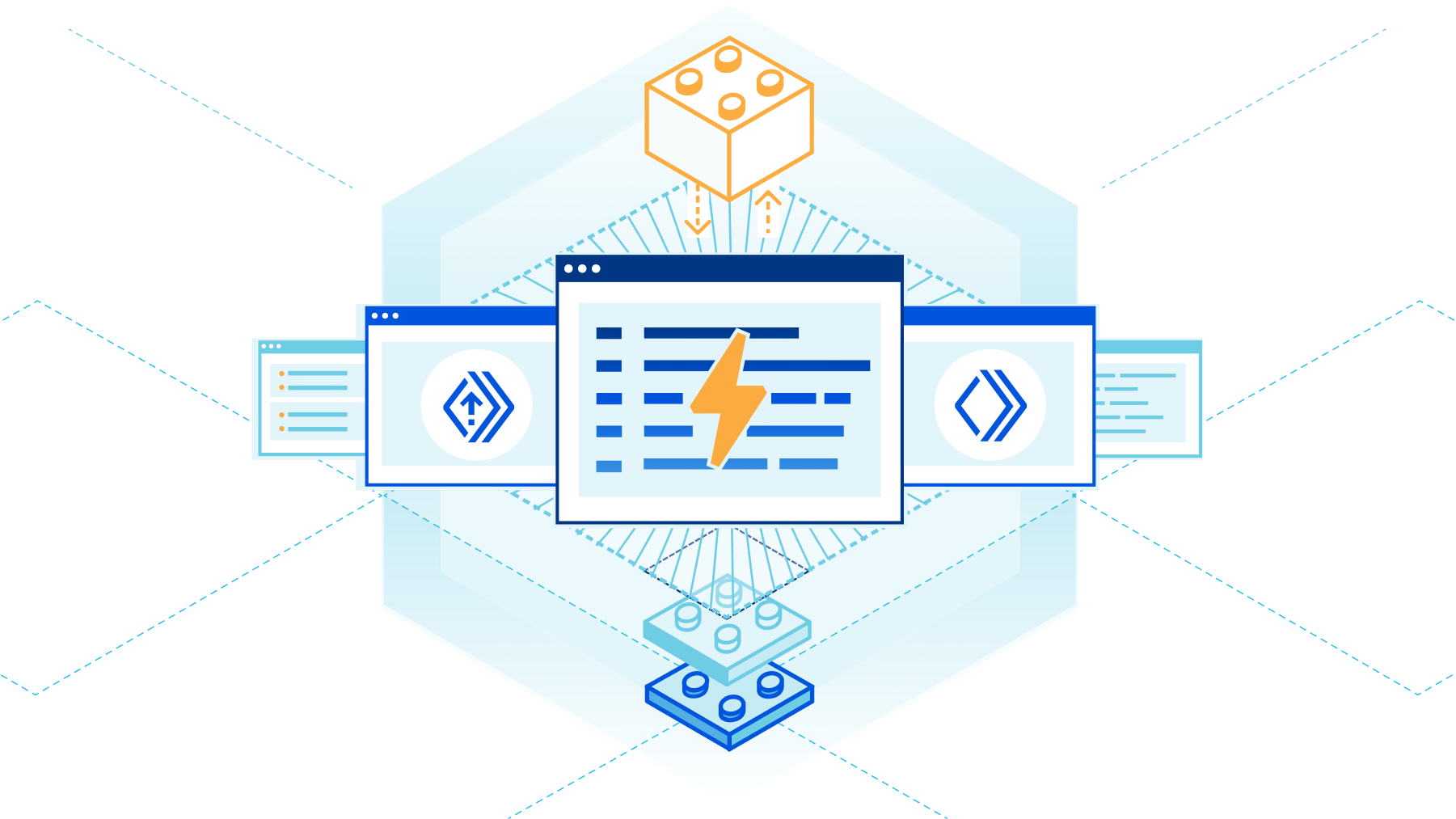
On the Cloudflare Developer Platform, we understand that building any application is a unique experience for every developer. We know that in the developer ecosystem there are a plethora of tools to choose from and as a developer you have preferences and needs. We don’t believe there are “right” or “wrong” tools to use in development and want to ensure a good developer experience no matter your choices. We believe in meeting you where you are.
When Pages Functions moved to Generally Available in November of last year, we knew it was the key that unlocks a variety of use cases – namely full-stack applications! However, we still felt we could do more to provide the flexibility for you to build what you want and how you want.
That’s why today we’re opening the doors to developers who want to build their server side applications with something other than JavaScript. We’re excited to announce WebAssembly support for Pages Functions projects!
WebAssembly (or Wasm) is a low-level assembly-like language that can run with near-native performance. It provides programming languages such as C/C++, C# or Rust with a compilation target, enabling them to run alongside JavaScript. Primarily designed to run on the web (though not exclusively), WebAssembly opens up exciting opportunities for applications to run on the web platform, both on the client and the server, that up until now couldn't have done so.
With Pages Functions being Workers “under the hood” and Workers having Wasm module support for quite some time, it is only natural that Pages provides a similar experience for our users as well. While not all use cases are a good fit for Wasm, there are many that are. Our goal with adding Wasm support is enabling those use cases and expanding the boundaries of what Functions can build.
Using WebAssembly in Pages Functions
WebAssembly in Pages Functions works very similar to how it does today in Workers — we read wasm
files as WebAssembly modules, ready for you to import and use directly from within your Functions. In short, like this:
// functions/api/distance-between.js
import wasmModule from "../../pkg/distance.wasm";
export async function onRequest({ request }) {
const moduleInstance = await WebAssembly.instantiate(wasmModule);
const distance = await moduleInstance.exports.distance_between();
return new Response(distance);
}
Let’s briefly unpack the code snippet above to highlight some things that are important to understand.
import wasmModule from "../../pkg/distance.wasm";
Pages makes no assumptions as to how the binary .wasm
files you want to import were compiled. In our example above, distance.wasm
can be a file you compiled yourself out of code you wrote, or equally, a file provided in a third-party library’s distribution. The only thing Pages cares about is that distance.wasm
is a compiled binary Wasm module file.
The result of that import is a WebAssembly.Module
object, which you can then instantiate:
const moduleInstance = await WebAssembly.instantiate(wasmModule);
Once the WebAssembly.Instance
object is created, you can start using whatever features your Wasm module exports
, inside your Functions code:
const distance = await moduleInstance.exports.distance_between();
More modules, more fun!
Apart from Wasm modules, this work unlocks support for two other module types that you can import within your Functions code: text and binary. These are not standardized modules, but can be very handy if you need to import raw text blobs (such as HTML files) as a string
:
// functions/my-function.js
import html from "404.html";
export async function onRequest() {
return new Response(html,{
headers: { "Content-Type": "text/html" }
});
}
or raw data blobs (such as images) as an ArrayBuffer
.
// functions/my-function.js
import image from "../hearts.png.bin";
export async function onRequest() {
return new Response(image,{
headers: { "Content-Type": "image/png" }
});
}
The distance between us on the surface of Earth
Let’s take a look at a live example to see it all in action! We’ve built a small demo app that walks you through an example of Functions with WebAssembly end-to-end. You can check out the code of our demo application available on GitHub.
The application computes the distance in kilometers on the surface of Earth between your current location (based on the geo coordinates of the incoming request) and any other point on the globe, each time you click on the globe's surface.
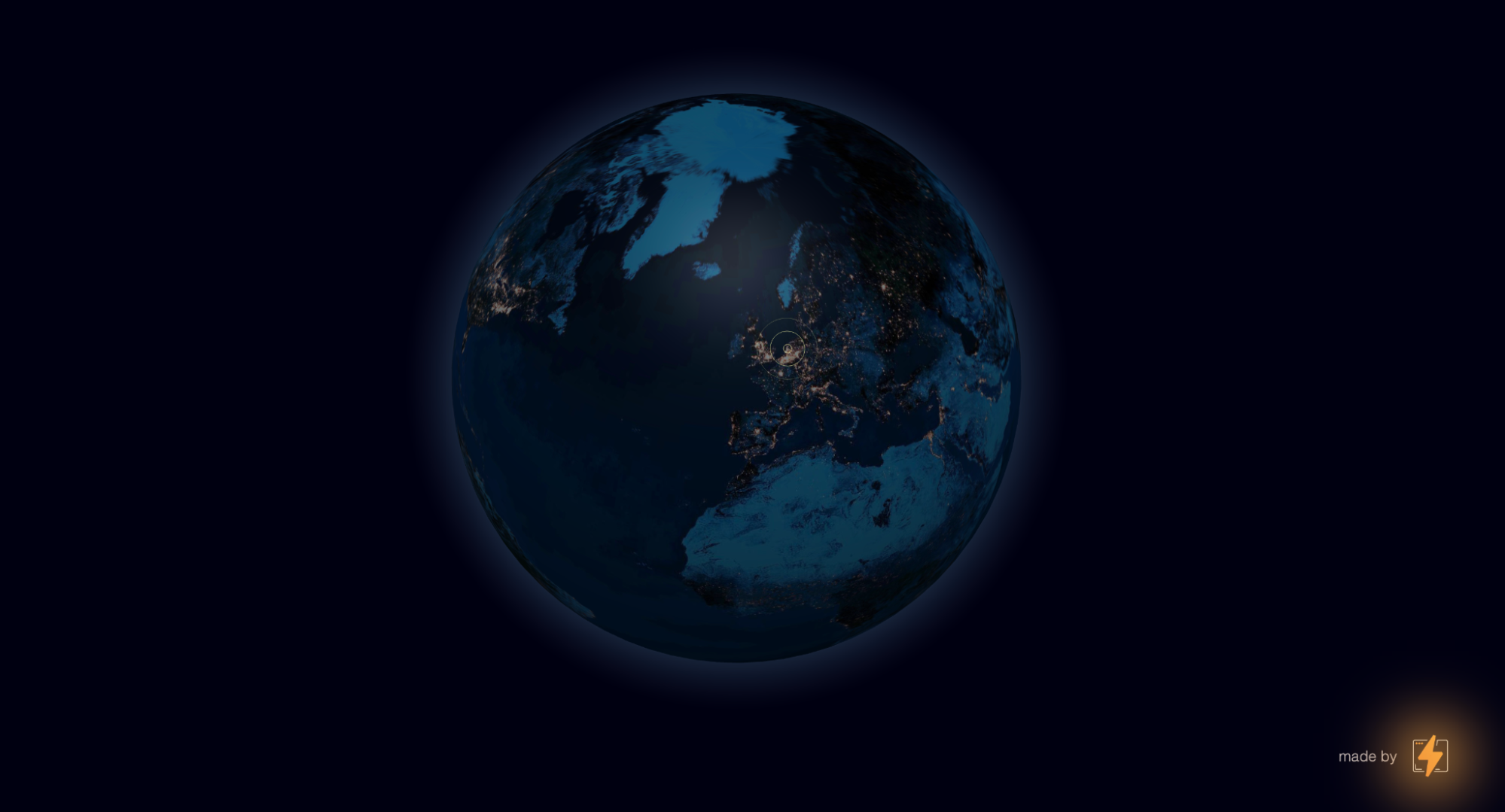
The code that performs the actual high-performance distance calculation is written in Rust, and is a slight adaptation of the example provided in the Rust cookbook:
fn distance_between(from_latitude_degrees: f64, from_longitude_degrees: f64, to_latitude_degrees: f64, to_longitude_degrees: f64) -> f64 {
let earth_radius_kilometer = 6371.0_f64;
let from_latitude = from_latitude_degrees.to_radians();
let to_latitude = to_latitude_degrees.to_radians();
let delta_latitude = (from_latitude_degrees - to_latitude_degrees).to_radians();
let delta_longitude = (from_longitude_degrees - to_longitude_degrees).to_radians();
let central_angle_inner = (delta_latitude / 2.0).sin().powi(2)
+ from_latitude.cos() * to_latitude.cos() * (delta_longitude / 2.0).sin().powi(2);
let central_angle = 2.0 * central_angle_inner.sqrt().asin();
let distance = earth_radius_kilometer * central_angle;
return distance;
}
We have a Rust playground experiment available here, in case you want to play around with this code snippet in particular.
To use the distance_between()
Rust function in Pages Functions, we first compile the code to WebAssembly using wasm-pack
:
##
# generate the `pkg` folder which will contain the wasm binary
##
wasm-pack build
Then, we import the generated .wasm
artifact from inside our distance-between.js
Pages Function. Now, each time you click on the globe surface, a request to /api/distance-between
is made, which will trigger the distance_between()
function to execute. Once computed, the distance value is returned by our Function, back to the client, which proceeds to display the value to the user.
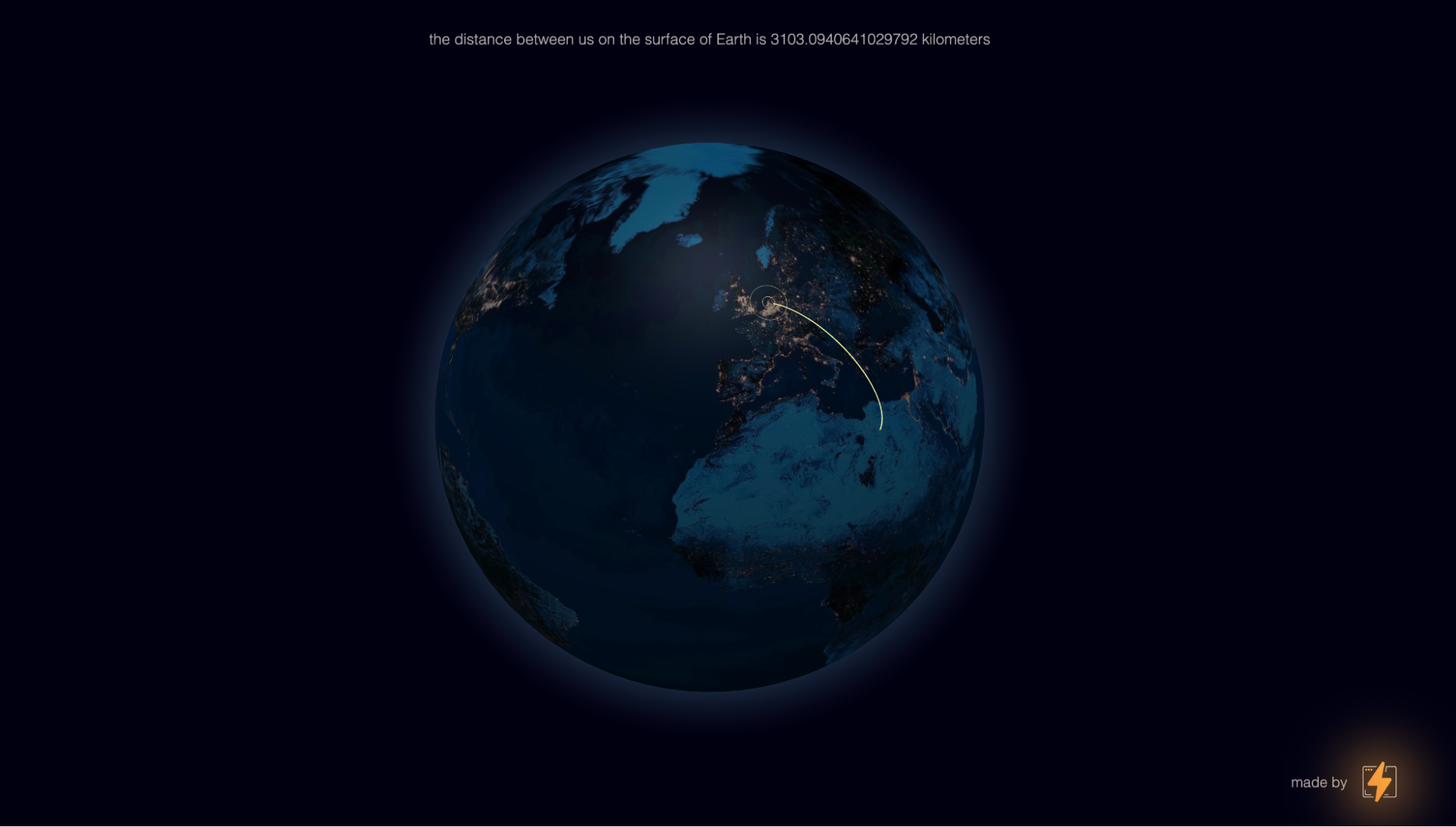
We want to point out that this application could have been built entirely in JavaScript, however, we equally wanted to show just how simple it is to build it with Rust. The decision to use Rust was motivated by a few factors. First, the tooling ecosystem for building and working with Rust-generated WebAssembly is quite mature, well documented, and easy to get started with. Second, the Rust docs are a fantastic resource if you are new to Rust or to Rust with WebAssembly! If you are looking for a step-by-step tutorial on how to generate and set up a Rust and WebAssembly project, we highly recommend checking out Rust’s official WebAssembly Book.
We hope it gives you a solid starting point in exploring what is possible with Wasm on Pages Functions, and inspires you to create some powerful applications of your own. Head over to our docs to get started today!